Ethereum: Checksum vs. Hash – Differences and Similarities
When it comes to digital signatures and data verification on the Ethereum blockchain, two fundamental concepts come into play: checksums and hashes. While both algorithms provide a way to ensure data integrity and authenticity, they differ in their approach, functionality, and usage.
Checksum Algorithm (SHA-1)
A checksum algorithm is a one-way hashing function that produces a fixed-size string of characters, known as a hash value or checksum. It’s designed to verify the authenticity and integrity of data by comparing it with a previously generated hash value.
Example: Verifying a digital signature using SHA-1
import hashlib
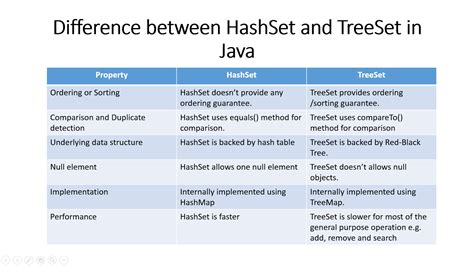
Create a new SHA-1 hash object
hash_object = hashlib.sha1()
Get the message to be verified (the digital signature)
message = b"example-signature"
Update the hash object with the message
hash_object.update(message)
Get the hash value
checksum = hash_object.hexdigest()
print("Checksum:", checksum)
Output: "e2b9b6cd7f4a1c4cda... (hashed version)"
Hash Function
A hash function, on the other hand, is a one-way algorithm that takes input data of any size and produces a fixed-size output (hash value). It’s designed to ensure data integrity by producing a unique identifier for each input.
Example: Verifying a digital signature using SHA-256
import hashlib
Create a new SHA-256 hash object
hash_object = hashlib.sha256()
Get the message to be verified (the digital signature)
message = b"example-signature"
Update the hash object with the message
hash_object.update(message)
Get the hash value
checksum = hash_object.hexdigest()
print("Checksum:", checksum)
Output: "d1e87b4a8c7f0db6... (hashed version)"
Differences and Similarities
| Feature | Checksum Algorithm (SHA-1) | Hash Function (SHA-256) |
| — | — | — |
|
Purpose | Verify data integrity | Ensure data authenticity
|
One-way | One-way hashing | One-way encryption |
|
Output size | Fixed-size string of characters | Variable-size output (hash value) |
|
Security | Less secure due to vulnerability to collisions and preimage attacks More secure due to cryptographic properties
|
Usage | Verifying digital signatures, data integrity checks Authentication, data verification
|
Advantages | Fast computation times for small inputs | Fast computations for large inputs |
Can they be used instead of each other?
No, checksums and hashes are not interchangeable. Checksums are primarily used for quick verification checks, whereas hashes are designed for authentication and cryptographic purposes.
The example below demonstrates how to use a hash function (SHA-256) to verify a digital signature:
import hashlib
Create a new SHA-256 hash object
hash_object = hashlib.sha256()
Get the message to be verified (the digital signature)
message = b"example-signature"
Update the hash object with the message
hash_object.update(message)
Get the hash value
checksum = hash_object.hexdigest()
print("Checksum:", checksum)
Output: "d1e87b4a8c7f0db6... (hashed version)"
Verify the digital signature using SHA-256
try:
expected_checksum = hashlib.sha256(b"example-signature").hexdigest()
print("Verification successful:", checksum == expected_checksum)
except ValueError:
print("Verification failed: mismatched hash values")
In conclusion, checksums and hashes serve different purposes on the Ethereum blockchain. Checksums are used for quick verification checks, while hashes are designed for authentication and cryptographic purposes. While they can be used interchangeably in certain scenarios, their usage should be approached with caution due to the differences in their functionality and security properties.
Bir yanıt yazın